[Ch3-7] Fragment, 프래그먼트란 무엇인고~? 간단하게 만들어 보기 (Using Fragments in Android)
Android Studio 공부 시작 2019. 7. 18. 23:48안드로이드의 액티비티(Activity), 뷰(View)와 더불어 중요 요소 중 하나인 프래그먼트(Fragments)를 배워보자!
Fragments 란?
- 모바일이나 태블릿에서 화면을 구성할 때, 많은 View(텍스트, 텍스트박스, 버튼)들이 사용되는데, 이를 정리하기 위하여 화면을 쪼개는 것이다.
지난번과 마찬가지로, 우리가 개발할 목표를 보고 난 뒤, 코드를 보도록 하자.
우리의 목표 : 프래그먼트를 사용하여 화면을 나눠보자!
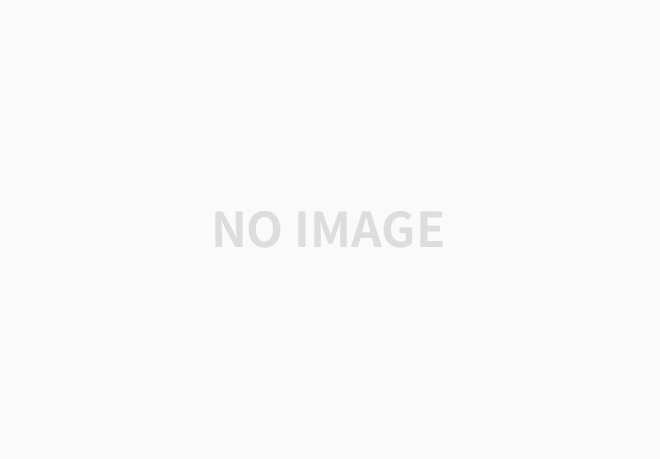
작업 준비물 : 신규 프로젝트 생성 -> Empty Project (프로젝트 명 : Fragments)
1. Fragment #1 만들기!
1.1 res -> layout 에서 폴더를 우클릭 후 New -> Layout resource file 클릭 후 "fragment1.xml"(이름 입력 시 ".xml"까지 포함)
코드 기입
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#00FF00"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="이건 프래그먼트 #1 임 ㅋ"
android:textColor="#000000"
android:textSize="25sp" />
</LinearLayout>
특별한 내용은 없다. TextView 는 단순히 이 프래그먼트가 몇번째인지를 알기 쉽게 하기 위하여 적어 놓았다.
다만 일반적인 layout 과는 다르게, 현재 LinearLayout 으로 정의되어 있음을 확인하고 넘어가자.
2. Fragment #2 만들기!
2.1 res -> layout 에서 폴더를 우클릭 후 New -> Layout resource file 클릭 후 "fragment2.xml"(이름 입력 시 ".xml"까지 포함)
코드 기입
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#FFFE00"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="이건 프래그먼트 #2임 ㅋ"
android:textColor="#000000"
android:textSize="25sp"/>
</LinearLayout>
마찬가지로 색과 글자만 바꾸었다.
3. Fragment1.java 만들기
코드부터 보자
package com.example.fragments;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
public class Fragment1 extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater,
ViewGroup container, Bundle savedInstanceState) {
// -- Inflate the layout for this fragment --
return inflater.inflate(
R.layout.fragment1, container, false);
}
}
일반적인 상황과 달라진 점을 살펴보자. 가장 대두적인 것은 import android.view.LayoutInflater; 구문이 추가되었으며 return 시 inflater 를 사용한다.
inflater는 무엇인가?
- 내가 이해한 바로는 layout을 담고 있는 xml 파일을 읽어오며 그 파일이 포함하고 있는 object 까지 읽어온다는 기능이다. 이름이 inflate(부풀리다)인 이유는, layout을 불러올 때, 적용할 xml 파일 (우리의 경우는 activity_main.xml)에 맞춰 layout을 팽창하여 적용시키기 때문이지 않을까? (혹시 추가 설명이나 잘못된 점이 있다면 답글 부탁드립니다.)
4. Fragment2.java 만들기
package com.example.fragments;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
public class Fragment2 extends Fragment {
@Override
public View onCreateView (LayoutInflater inflater,
ViewGroup container, Bundle savedInstanceState) {
// -- Inflate the layout for this fragment --
return inflater.inflate(
R.layout.fragment2, container, false);
}
}
마찬가지로, Fragment1.java 와 같다. 이름이 바뀌었음을 주의하자.
5. activity_main.xml 에서 Fragment 1과 Fragment 2 사용하기
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.example.fragments.MainActivity">
<fragment
android:name="com.example.fragments.Fragment1"
android:id="@+id/fragment1"
android:layout_weight="1"
android:layout_width="fill_parent"
android:layout_height="match_parent" />
<fragment
android:name="com.example.fragments.Fragment2"
android:id="@+id/fragment2"
android:layout_weight="1"
android:layout_width="fill_parent"
android:layout_height="match_parent"
/>
</LinearLayout>
※ @dimen 쪽에서 명시되지 않았다고 에러를 띄워줄텐데, 일단은 그냥 넘어가자.
여기는 그냥 Fragment 1과 2를 정의하는 구간이다.
자! 이제 실행해보자.
error: resource dimen/activity_vertical_margin (aka com.example.fragments:dimen/activity_vertical_margin) not found.
어떠한가? @dimen 쪽에서 위와 같은 에러가 발생하는가?
우리가 @dimen 을 정의하지 않았음으로 당연히 에러가 발생한다. 그럼 어떻게 해야하나??
간단히 정의하면 된다.
1. res -> values -> 폴더 우클릭 후 -> values resource file 클릭한다.
2. "dimens.xml" 이름으로 xml 파일을 생성한다.
3. 아래의 코드 입력
<?xml version="1.0" encoding="utf-8"?>
<resources>
<dimen name="activity_vertical_margin">4dp</dimen>
<dimen name="activity_horizontal_margin">4dp</dimen>
</resources>
다음과 같이 정의하면 @dimen 으로 미리 정의된 크기의 마진을 사용할 수 있다.
이제 다시 실행해보면 에러가 안날것이다.
다들 모두 수고했어용~